Scripted Access via TAP
This web application is an instance of Daiquiri, which comes with a built-in TAP interface according to the specifications from the International Virtual Observatory Alliance (IVOA).
The Table Access Protocol (TAP) was developed by IVOA is a second generation DAL interface being developed to provide a general access mechanism for tabular data, including but not limited to astronomical catalogs.
The URL for the APPLAUSE TAP service is: https://www.plate-archive.org/tap/
You can find the full TAP documentation on the IVOA documentation website.
How to use TAP in Python
To run the code examples below, you will need Python 3 and pyvo version>=1.0.
pip install pyvo
Pyvo allows to set a token for authorized access to your user account.
Your Daiquiri token is accessible at https://www.plate-archive.org/accounts/token/, once you're logged in. You'll need to replace the token of the examples with your own .
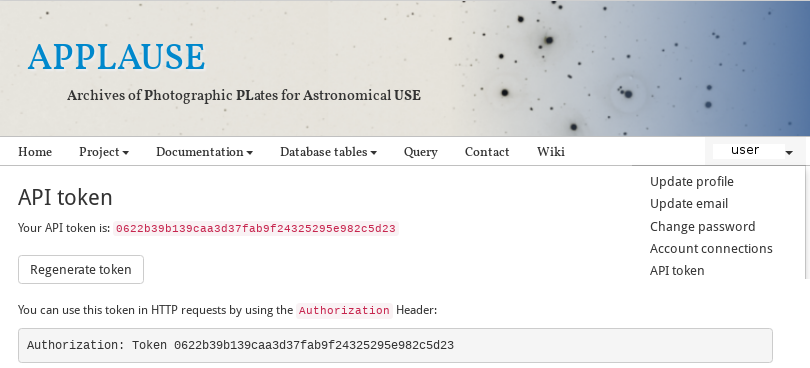
This is an example in Python for accessing the DR3 archive table and printing the name of each archive.
import requests
import os.path
import pyvo as vo
import numpy as np
import pandas
name = 'APPLAUSE',
url = 'https://www.plate-archive.org/tap'
token = 'Token YOURTOKEN'
qstr = 'SELECT * FROM applause_dr3.archive limit 5'
print('\npyvo version %s \n' % vo.__version__)
print('TAP service %s \n' % name)
tap_session = requests.Session()
tap_session.headers['Authorization'] = token
tap_service = vo.dal.TAPService(url, session=tap_session)
lang = 'PostgreSQL'
job = tap_service.submit_job(qstr, language=lang)
job.run()
job.wait(phases=["COMPLETED", "ERROR", "ABORTED"], timeout=30.)
job.raise_if_error()
results = job.fetch_result()
print(results)
The full documentation how to use TAP in Topcat is located here.
How to use TAP on the command line
TAP can also be used with a HTTP command line client. Here we use HTTPie, but there are a lot of other similar clients (e.g. curl).
As with the Python interface, you can also use your personal token to authenticate with the system to use your personal account (and your personal joblist, quota etc.). To do so, you need to send the token as part of the Authorization
header with every HTTP request.
http https://www.plate-archive.org/tap/async Authorization:"Token YOURTOKEN"
# retrieve the job list
http https://www.plate-archive.org/tap/async
# submit an asyncronous job (using PostgreSQL and the 5 minutes queue)
http -f --follow POST https://www.plate-archive.org/tap/async \
QUERY="SELECT raj2000, dej2000 FROM applause_dr3.source_calib LIMIT 10" \
LANG="postgresql-9.6" QUEUE="1h" PHASE="RUN"
# get all the information about a job
http https://www.plate-archive.org/tap/async/78d9c528-8cf0-46e3-8a5b-ec151229a30b
# check the status of a job
http https://www.plate-archive.org/tap/async/78d9c528-8cf0-46e3-8a5b-ec151229a30b/phase
# get the results of a job as csv or votable
http https://www.plate-archive.org/tap/async/78d9c528-8cf0-46e3-8a5b-ec151229a30b/results/csv
http https://www.plate-archive.org/tap/async/78d9c528-8cf0-46e3-8a5b-ec151229a30b/results/votable
# archive the job (this deletes the database table and frees up space)
http --follow DELETE https://www.plate-archive.org/tap/async/78d9c528-8cf0-46e3-8a5b-ec151229a30b